有根树
有根树,顾名思义,就是有根节点的树结构。
有根树的节点之间存在父子关系,其中,有一个节点,叫做“根节点”。根节点没有父节点。

如图所示,1号节点就是根节点,它是唯一一个没有父节点的节点。
以节点5为例,它的兄弟节点分别是2、11,父节点为1,子节点分别为6、7、8.
我们把没有子节点的节点成为叶节点。
有根树中,节点x的子节点数被称为x的度,比如,节点5的度为3.
从根节点到节点x的路径长度称为x的深度,另外,节点x到叶节点的最大路径长度为节点x的高。一棵树中,根节点的高度最大,所以我们把根节点的高称为树的高。
那我们怎么存储这样一个数据结构呢?
自然而然地,我们会想到,把每个节点的父节点、各个子节点的信息都给保存下来,那不就可以把整个有根树保存下来了吗?
可是,这样就存在问题了。每个节点的子节点的数目不一定,那么我们在c++中定义数据结构的时候,就不是很方便。(当然,不得不吐槽的就是,cpp的代码又长又臭。如果是在python里面的话,用上面这种思路定义有根树是很方便的。)但是,如果想实现复杂度为O(N)的遍历,用上面的定义方式就不是很方便了。
那怎么办呢?
我们冷静分析一波:
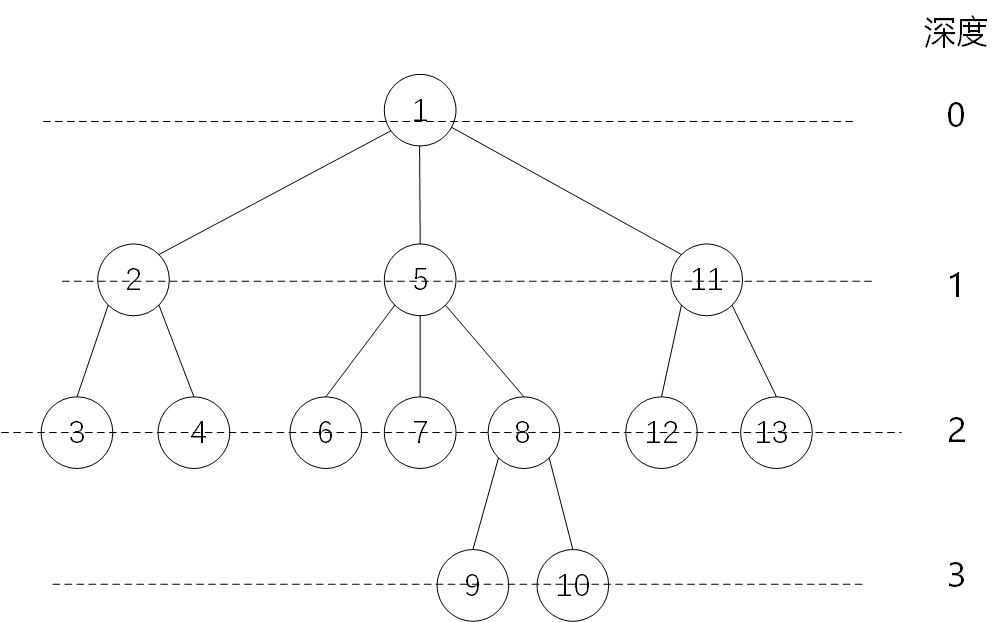
在上面这棵树里,如果我们想要知道每一个节点的确切位置,我们只需要存储当前节点的最左侧子节点和紧邻的右兄弟节点即可确定当前节点在整个树中的位置。
这就是“左子右兄弟表示法”,根据这个方法,我们能通过遍历得到每一个节点的位置信息。
题目
题目位于
http://judge.u-aizu.ac.jp/onlinejudge/description.jsp?id=ALDS1_7_A
因为题目太长然后机器翻译太不准,就不人工翻译写到这了,我就直接把英文原题贴上来吧。
Your task is to write a program which reports the following information for each node u of a given rooted tree T:
node ID of u
parent of u
depth of u
node type (root, internal node or leaf)
a list of chidlren of u
If the last edge on the path from the root r of a tree T to a node x is (p, x), then p is the parent of x, and x is a child of p. The root is the only node in T with no parent.
A node with no children is an external node or leaf. A nonleaf node is an internal node
The number of children of a node x in a rooted tree T is called the degree of x.
The length of the path from the root r to a node x is the depth of x in T.
Here, the given tree consists of n nodes and evey node has a unique ID from 0 to n-1.
Input
The first line of the input includes an integer n, the number of nodes of the tree.
In the next n lines, the information of each node u is given in the following format:
id k c1 c2 … ck
where id is the node ID of u, k is the degree of u, c1 … ck are node IDs of 1st, … kth child of u. If the node does not have a child, the k is 0.
Output
Print the information of each node in the following format ordered by IDs:
node id: parent = p, depth = d, type, [c1…ck]
p is ID of its parent. If the node does not have a parent, print -1.
d is depth of the node.
type is a type of nodes represented by a string (root, internal node or leaf). If the root can be considered as a leaf or an internal node, print root.
c1…ck is the list of children as a ordered tree.
Please follow the format presented in a sample output below.
Constraints
1 ≤ n ≤ 100000
Sample Input 1
13
0 3 1 4 10
1 2 2 3
2 0
3 0
4 3 5 6 7
5 0
6 0
7 2 8 9
8 0
9 0
10 2 11 12
11 0
12 0
Sample Output 1
node 0: parent = -1, depth = 0, root, [1, 4, 10]
node 1: parent = 0, depth = 1, internal node, [2, 3]
node 2: parent = 1, depth = 2, leaf, []
node 3: parent = 1, depth = 2, leaf, []
node 4: parent = 0, depth = 1, internal node, [5, 6, 7]
node 5: parent = 4, depth = 2, leaf, []
node 6: parent = 4, depth = 2, leaf, []
node 7: parent = 4, depth = 2, internal node, [8, 9]
node 8: parent = 7, depth = 3, leaf, []
node 9: parent = 7, depth = 3, leaf, []
node 10: parent = 0, depth = 1, internal node, [11, 12]
node 11: parent = 10, depth = 2, leaf, []
node 12: parent = 10, depth = 2, leaf, []
Sample Input 2
4
1 3 3 2 0
0 0
3 0
2 0
Sample Output 2
node 0: parent = 1, depth = 1, leaf, []
node 1: parent = -1, depth = 0, root, [3, 2, 0]
node 2: parent = 1, depth = 1, leaf, []
node 3: parent = 1, depth = 1, leaf, []
求节点深度
求节点u的深度的时候,我们可以通过递归来求深度,那么复杂度就会降低到O(n)
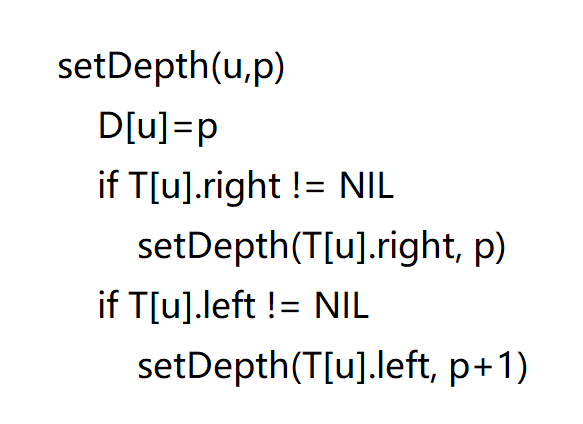
代码实现
#include<iostream>
#include<cstdio>
#include<string>
using namespace std;
#define MAX 100000
#define NIL -1
struct Node
{
int parent;
int left_son;
int right_brother;
};
Node T[MAX];
int depth[MAX], son[MAX];
int n;
void Searching(int id, int dp)
{
depth[id] = dp;
if(T[id].right_brother!=NIL)
Searching(T[id].right_brother, dp);
if(T[id].left_son!=NIL)
Searching(T[id].left_son, dp+1);
}
void print_son(int id)
{
cout<<" [";
int tmp = T[id].left_son;
while(tmp!=-1)
{
cout<<tmp;
if(T[tmp].right_brother != -1) cout<<", ";
tmp = T[tmp].right_brother;
}
cout<<"]"<<endl;
}
void print()
{
for(int i=0;i<n;i++)
{
string status=" ";
if(T[i].parent == -1) status = "root";
else if(T[i].left_son == -1) status = "leaf";
else status = "internal node";
printf("node %d: parent = %d, depth = %d, ",i,T[i].parent, depth[i]);
cout<<status<<",";
print_son(i);
}
}
int main()
{
cin>>n;
//初始化所有的节点
for(int i=0;i<n;i++)
{
T[i].parent = NIL;
T[i].left_son = NIL;
T[i].right_brother = NIL;
}
int k, last_father = NIL;
for(int i=0;i<n;i++)
{
cin>>k;
int du;
cin>>du;
if(du==0) continue;
int tmp;
int last_left = NIL;
for(int j=0;j<du;j++)
{
cin>>tmp;
T[tmp].parent = k;
if(j==0)
{
last_left = tmp;
T[k].left_son = tmp;
continue;
}
T[last_left].right_brother = tmp;
last_left = tmp;
}
}
int root;
for(int i=0;i<n;i++)
if(T[i].parent==-1) root = i;
Searching(root, 0);
print();
}
欢迎关注我的公众号“灯珑”,让我们一起了解更多的事物~
